Scenario
Let's say you have many Views that share the same layout but the information in them are different,
kinda like a blog or news feed. It'd be nice to have Structured Data dynamically built based on
what you pull from a data source, assuming you have a data source. I'mma try to walk you through the
process.
This is my story.
Overview
In this snippet I will walk you through the process of dynamically building Stuctured Data in JSON-LD format in MVC ASP.NET for an Article. We will be using some C# and some Razor syntax to build the Stuctured Data in our View. For this example I will assume you have a data source and I'll be using a simple list that I'll pass to the view.
Straight Into It
I've chosen the Snippet format because a full length, start to end article would've been too long. So I'll be making a few assumptions here and there like you having access to a data source, however basic as it may be (it could be a plain ol' like the one I'll be using). I'll still try to give as much context and information as possible.
Model
First we'll update or create a Model that will be responsible for your Article. This Model will need to to have recommened properties as described on Google's guide on Structured Data for Articles. So:
- dateModified
- datePublished
- headline
- image
There's more than just these four but fortunately enough these are the four that are most likely to be subject to change across views.
There's more properties that could lead to Rich Cards being enabled by Google which is nice.
Google says:
"The more recommended properties that you provide, the higher quality the result is to users.
For example: users prefer job postings with explicitly stated salaries than those without; users prefer recipes
with actual user reviews and genuine star ratings (note that reviews or ratings not by actual users are considered
spammy).
Rich result ranking takes extra information into consideration."
We're looking at "NON-AMP" compatible Structured Data because it requires sooooo much less set up, or at least from what I've seen but you're more than free to use Structured Data compatible with AMP sites.
Add these lines to your Model:
- public DateTime dateModified { get; set; }
- public DateTime datePublished { get; set; }
- public string headline { get; set; }
- public string image { get; set; }
Controller
In the Controller that will be handling the passing of your Model's data to your View, be sure to
populate the four properties we've declared with the relevant data. dateModified
and
datePublished
need to adhere to
ISO 8601
format. You can do the date conversion in the Controller or you can do the conversion in the View.
In the Controller it will look something like this:
[Your_Modal_Here].dateModified.ToString("s", System.Globalization.CultureInfo.InvariantCulture)
and the same goes for datePublished
.
View
In your View's <head>
tag we'll be adding the <script>
tag that will
house our structured data.
Below is an example of what I had as my structured data when I had written this article:
- <script type="application/ld+json">
- {
- "@@context": "http://schema.org",
- "@@type": "Article",
- "mainEntityOfPage": {
- "@@type": "WebPage",
- "@@id": "[The URL of where the article can be found]"
- },
- "headline": "@Model.headline",
- "image": {
- "@@type": "ImageObject",
- "url": "@Model.image"
- },
- "datePublished": "@Model.datePublished.ToString("s", System.Globalization.CultureInfo.InvariantCulture)",
- "dateModified": "@Model.dateModified.ToString("s", System.Globalization.CultureInfo.InvariantCulture)",
- "author": {
- "@@type": "Person",
- "Name": "[Your or the author's name here]"
- },
- "publisher": {
- "@@type":"Organization",
- "name":"[Name of your publisher here]",
- "logo":{
- "@@type": "ImageObject",
- "url": "[Your publisher's logo URL here]"
- }
- },
- "thumbnailUrl": "[Your article's thumbnail URL here]"
- }
- </script>
Replace the values in the square backets with the relevent information. These values can also be handled
with the a Model, just add as many properties as you see fit. A more fleshed out Structured Data set is
surely more preferred but be sure to stick to guidelines. Google has an
example that you should look at.
Also notice that in lines 14 and 15 I've done the coversion to ISO 8601, where you decide to do the formatting is up to you.
You're able to test the validity of any sites Structured Data here and you can even take the URL of this page and test it. And you're even able to test whether your Structured Data supports Rich Results here.
Here's what the Structured Data for this article looks like when tested in the Structured Data Testing Tool:
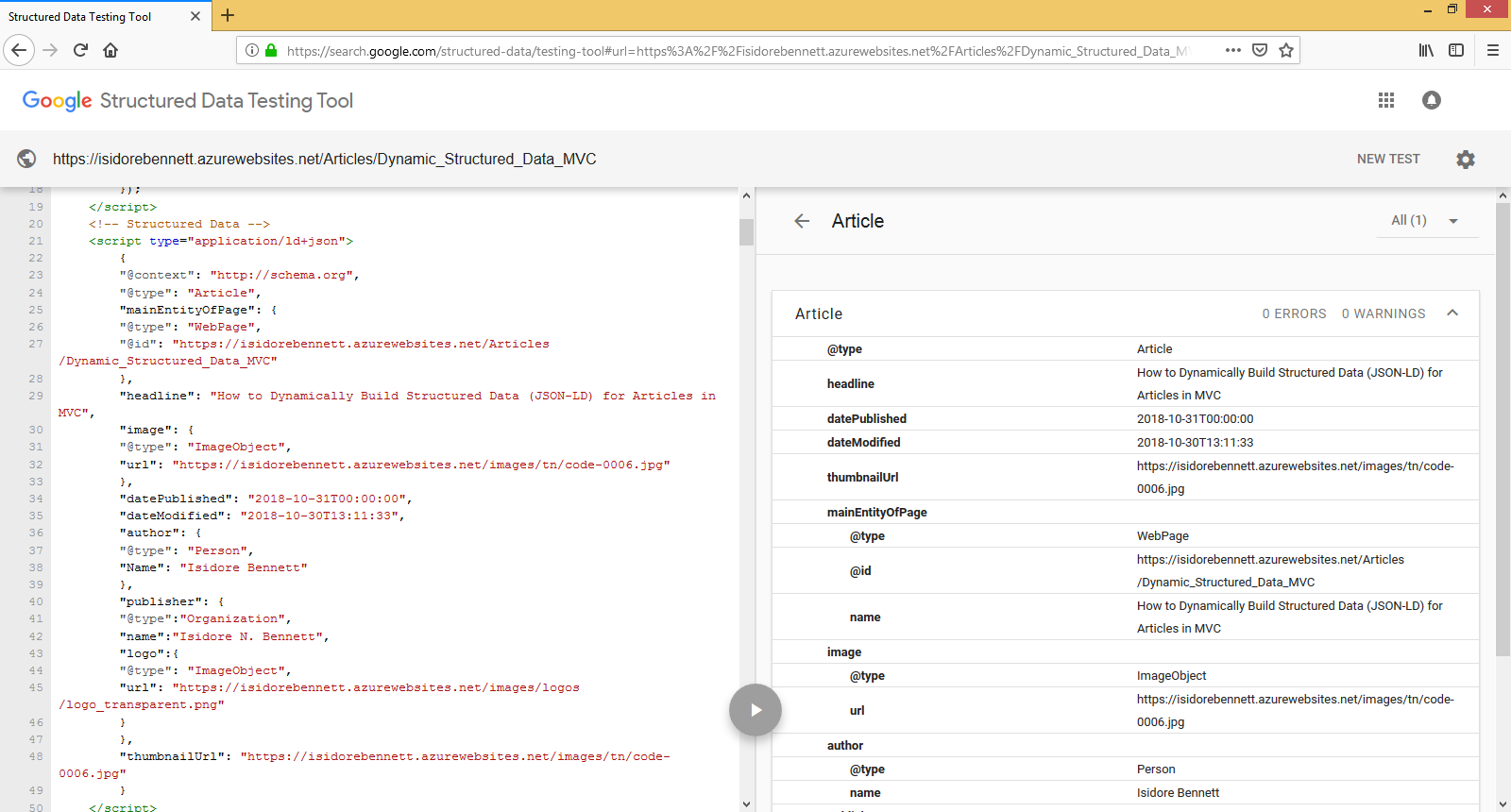
Don't mind the dates being swapped around too much loool, it's a bug that needs fixing:P
Thanks for reading.